Settings Tutorial
As discussed in the Settings Guide, plugins can add Settings to IntelliJ Platform-based IDEs. The IDE displays the Settings in response to a user choosing . Custom Settings are displayed and function like those native to the IDE.
Overview of Custom Settings Implementation
Using the SDK code sample settings
, this tutorial illustrates the steps to creating custom application-level settings. Many IntelliJ Platform Settings implementations use fewer classes, but the settings
code sample factors the functionality into three classes for clarity:
The
AppSettingsConfigurable
is analogous to a Controller in the MVC model – it interacts with the other two Settings classes and the IntelliJ Platform,The
AppSettings
is like a Model because it stores the Settings persistently,The
AppSettingsComponent
is similar to a View because it displays and captures edits to the values of the Settings.
The structure of the implementation is the same for Project Settings, but there are minor differences in the Configurable
implementation and extension point (EP) declaration.
The AppSettings
Class
The AppSettings
class persistently stores the custom Settings. It is based on the IntelliJ Platform Persistence Model.
Declaring AppSettings
Given a Light Service is not used, the persistent data class must be declared as a Service EP in the plugin.xml file. If these were Project Settings, the com.intellij.projectService
EP would be used. However, because these are Application Settings, the com.intellij.applicationService
EP is used with the fully qualified name (FQN) of the implementation class:
Creating the AppSettings
Implementation
As discussed in Implementing the PersistentStateComponent Interface, AppSettings
uses the pattern of implementing PersistentStateComponent
parameterized by a separate state class:
@Storage
Annotation
The @State
annotation, located above the class declaration, defines the data storage location. For AppSettings
, the data name
parameter is the FQN of the class. Using FQN is the best practice to follow and is required if custom data gets stored in the standard project or workspace files.
The storages
parameter uses the @Storage
annotation to define a custom filename for the AppSettings
data. In this case, the file is located in the options
directory of the configuration directory for the IDE.
Persistent State Class
The AppSettings
implementation contains an inner state class with two public fields: a String
and a boolean
. Conceptually, these fields hold the name of a user and whether that person is an IntelliJ IDEA user, respectively. See Implementing the State Class for more information about how PersistentStateComponent
serializes public fields.
AppSettings
Methods
The fields are so limited and straightforward for this class that encapsulation is not used for simplicity. All that is needed for functionality is to override the two methods called by the IntelliJ Platform when a new component state is loaded (PersistentStateComponent.loadState()
), and when a state is saved (PersistentStateComponent.getState()
). See PersistentStateComponent
for more information about these methods.
One static convenience method has been added – AppSettings.getInstance()
– which allows AppSettingsConfigurable
to easily acquire a reference to AppSetting
.
The AppSettingsComponent
Class
The role of the AppSettingsComponent
is to provide a JPanel
for the custom Settings to the IDE Settings Dialog. The AppSettingsComponent
has-a JPanel
, and is responsible for its lifetime. The AppSettingsComponent
is instantiated by AppSettingsConfigurable
.
Creating the AppSettingsComponent
Implementation
The AppSettingsComponent
defines a JPanel
containing a JBTextField
and a JBCheckBox
to hold and display the data that maps to the data fields of AppSettings.State
:
AppSettingsComponent
Methods
The constructor builds the JPanel
using the convenient FormBuilder
and saves a reference to the JPanel
. The rest of the class are simple accessors and mutators to encapsulate the UI components used on the JPanel
.
The AppSettingsConfigurable
Class
The methods of AppSettingsConfigurable
are called by the IntelliJ Platform, and AppSettingsConfigurable
in turn interacts with AppSettingsComponent
and AppSettings
.
Declaring the AppSettingsConfigurable
As described in Declaring Application Settings, the com.intellij.applicationConfigurable
is used as the EP. An explanation of this declaration can be found in Declaring Application Settings:
Creating the AppSettingsConfigurable
Implementation
The AppSettingsConfigurable
class implements Configurable
. The class has one field to hold a reference to the AppSettingsComponent
.
AppSettingsConfigurable
Methods
All the methods in this class are overrides of the methods in the Configurable
interface. Readers are encouraged to review the Javadoc comments for the Configurable
methods. Also, review notes about IntelliJ Platform Interactions with Configurable
methods.
Testing the Custom Settings Plugin
After performing the steps described above, compile and run the plugin in a Development Instance to see the custom Settings available in the Settings Dialog. Open the IDE Settings by selecting
. The settings are preloaded with the default values: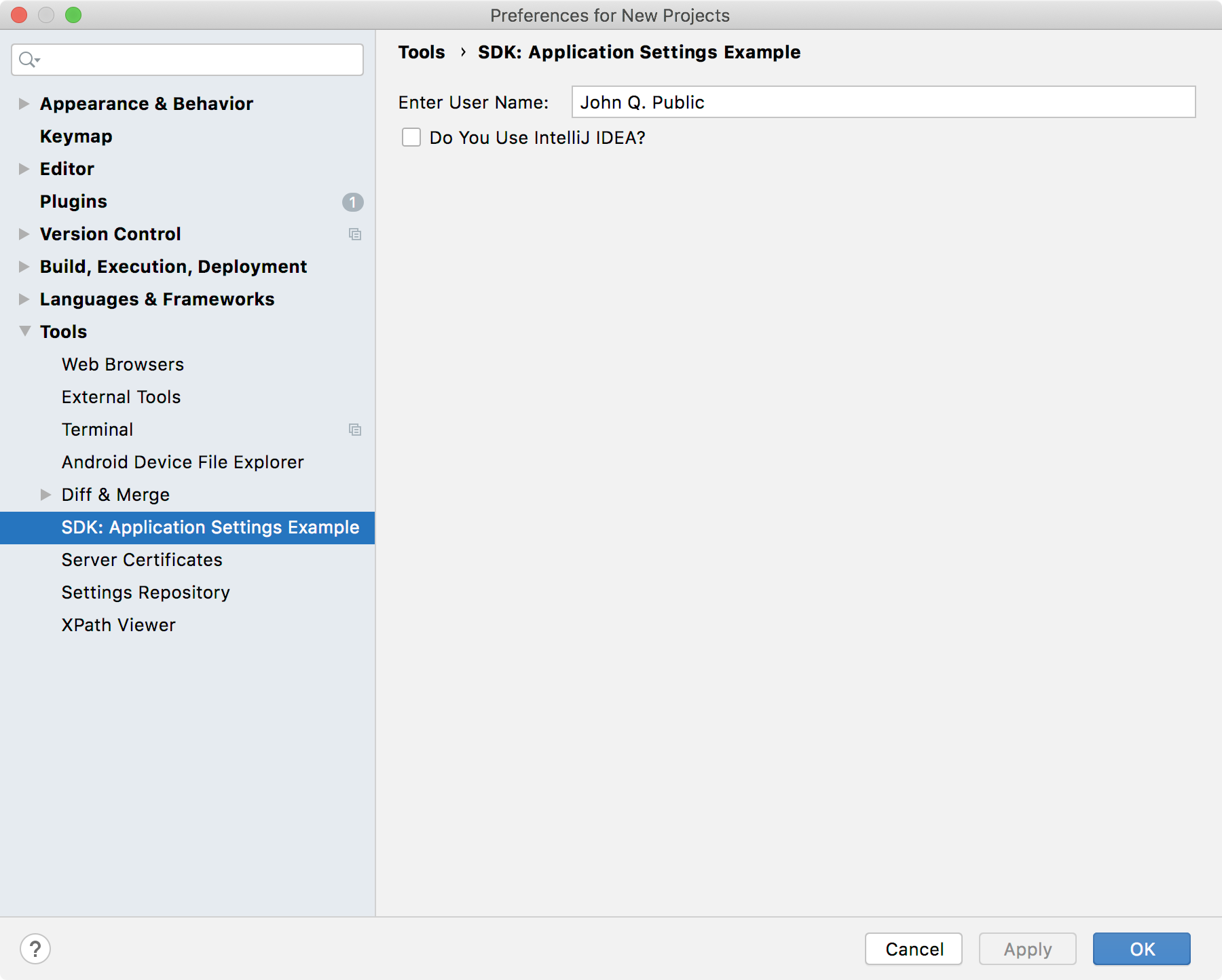
Now edit the settings values to "John Doe" and click the checkbox. Click the OK button to close the Settings dialog and save the changes. Exit the Development Instance.
Open the file code_samples/settings/build/idea-sandbox/config/options/SdkSettingsPlugin.xml to see the Settings persistently stored.