Explore the IntelliJ Platform API
Sometimes it can be challenging to implement plugin features for the IntelliJ Platform, especially when you've hit a roadblock, and you're unsure how to move forward. This usually happens in two situations:
You're trying to implement a feature that you've already seen in the IDE, and now you need to find the appropriate extension point or class that allows you to hook into the relevant mechanisms.
You've already started working on a feature, but you're unsure how the different parts of the IntelliJ Platform interact with each other. In such situations, it is helpful to be able to navigate the IntelliJ Platform code confidently and to find relevant examples in other plugins.
This guide provides a list of proven strategies that can help you overcome these challenges and gather enough information to continue your work. Furthermore, the tips below will help build your confidence as you explore the IntelliJ Platform.
1 Extension Points (EPs)
1.1 Browse Lists of EPs
The most important resource for discovering new EPs is the extensive list provided directly in the IntelliJ Platform SDK Documentation. On this page, you will find all the EPs, and each entry includes a link to the online source code and a link to the IntelliJ Platform Explorer, which helps you find examples of this EP in other plugins. Additionally, dedicated Extension Point Lists specific to IDEs are available under Product Specific.
1.2 Use Autocompletion Information
Another way to discover EPs is by using autocompletion or navigating through EP XML files. When you open a new tag in your plugin.xml file (inside the <extensions>
block with defaultExtensionNs="com.intellij"
), the IDE will automatically suggest possible EPs.
This is the first step in discovering new features that haven't been explicitly mentioned in the IntelliJ Platform Docs. Note that in the completion popup, you can call quick documentation, which then shows its properties, the implementation class, as well as a direct link to open usage results from IntelliJ Platform Explorer.
1.3 Search the IntelliJ Platform Code
Use Go to Declaration on EPs that are implemented in plugin.xml to navigate to its definition in the XML file. There you'll find more EPs, and browsing through this list helps you discover features you might not have been aware of. Search everywhere or Go to file helps you search for all files containing extension points. Just use *ExtensionPoints.xml as the search pattern and select the All Places scope.
However, if a bundled or third-party plugin exposes EPs for others to implement, these EPs are defined in the plugin.xml files of the plugins and not in the *ExtensionPoints.xml files of the IntelliJ Platform. One such example is the EPs exposed by the Markdown plugin that adds support for custom languages inside fenced code blocks of Markdown files.
1.4 Use Advanced Search
Explore the plugin.xml files of bundled or 3rd party plugins. If you have the IntelliJ Platform sources available either in your own plugin project or in a separate instance, you can use Structural Search to find all the files that meet the following criteria:
The file type is XML
It contains the tag
<idea-plugin>
The file is in the scope Project and Libraries
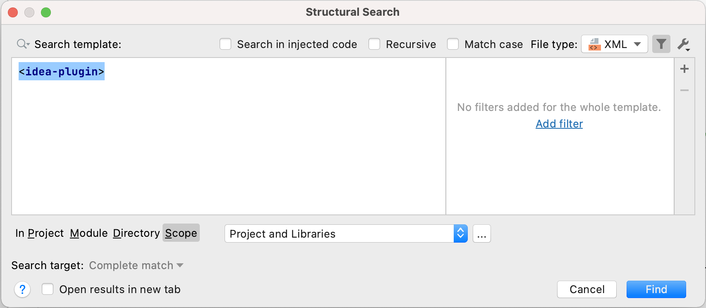
The search results will contain many plugin XML files. To find specific implementations of EPs in third-party plugins, use the IntelliJ Platform Explorer (see 3.2). Inspecting the plugin.xml files of other plugins not only helps you discover new features but also shows how things like menu entries or notification groups can be defined in the XML file.
2 Navigating the IntelliJ Platform Source Code
The following tips will help you navigate through the IntelliJ Platform source code if you already have an idea of what you're looking for. It's important that you're familiar with navigating and searching source code, as well as other basic features of IntelliJ IDEA.
Many developers keep the IntelliJ Community source code open in a separate IDE project while working on their plugin. Others search the source code of the IntelliJ Platform that is attached by default when using a Gradle-based project. While both methods work, it should be noted that developing plugins without inspecting the IntelliJ Platform code is nearly impossible, and all the tips below assume having the sources available.
2.1 Find Example Implementations
When working with interfaces or abstract classes of EPs, use IntelliJ IDEA's Go to Implementation or Find Usages feature to discover examples of how they are used in the IntelliJ Platform.
2.2 Look for Particular Class Names
Access to many features is provided through the Manager
and Service
classes, such as:
com.intellij.openapi.application.ApplicationManager
com.intellij.psi.PsiManager
Therefore, it can be helpful to search for classes that match the pattern com.intellij.*Manager
and look through the list of results. Note that not all of these classes have the com.intellij
prefix, and also that you can define custom scopes to limit your searches, for example, to only idea-xxx.jar files.
2.3 Inspect the Contents of Packages
If you open an EP's interface or abstract class, it is always helpful to inspect the contents of its package. For instance, the interface of the com.intellij.sdkType
EP lives in the com.intellij.openapi.projectRoots
package. Inspecting the contents of this package shows many related classes that will be useful if you are implementing this feature.
2.4 Search for Symbol Names
It is sometimes helpful to search directly for a method, class, and class member if you can guess a part of its name. You can either use Search Everything or Go to Symbol. Note that you need to change the search scope to All Places in the search window to find all occurrences of symbols.
2.5 Search by UI Text
If you want to implement a functionality that is similar to an existing IDE feature, but you can't guess the name of the extension point or implementation class, the underlying implementation can be found by the texts displayed in the UI.
Use the displayed text or its part as the target for a search within the IntelliJ Community project.
If the text is localized, this will identify a bundle file there the text is defined. Copy the key from the bundle file identified by the search.
Use the key text as the target for a search within the IntelliJ Community project. This search locates the implementation or related class, or plugin configuration file that uses the text key in an extension declaration.
If the key is found in the extension declaration in plugin.xml file, find the implementing class attribute value (in most cases it is
implementationClass
) and navigate to a declaration, or use attribute value as the target of a class search in the IntelliJ Community codebase to find the implementation.
If the text is not localized, the search will most probably find the desired implementation or related class. In this case, search for the found method/class usages, and repeat this until the actual implementation class is found.
2.6 Refrain from Using Internal Classes
As a general remark, the use of implementation classes is strongly discouraged (i.e., classes ending with Impl
in their name, located under impl
package, or included in *-impl.jar).
API annotated with @ApiStatus.Internal
must not be used, see Internal API Migration for more details and replacements.
3 Tools and References
3.1 Use Internal Mode and PsiViewer
When developing plugins, always enable the internal mode in IntelliJ IDEA. This provides access to a suite of tools to help you develop, debug, and test IntelliJ Platform plugins.
One of its most helpful features is the UI Inspector, which lets you investigate all parts of the UI of every IntelliJ-based IDE by simply clicking on them. Equally important is the tool. It will display all actions that are run by the IDE when you interact with UI elements, for example, by clicking a button.
Finally, the internal mode provides the PSI tree, please see documentation. The PsiViewer plugin is a separate plugin with similar capabilities for inspecting PSI trees, and it comes with a dedicated tool window that displays information on the fly. However, it does not display information about stubs or formatting models.
and actions, which allow you to analyze the3.2 Search the IntelliJ Platform Explorer
The IntelliJ Platform Explorer is a search tool for browsing Extension Points (EP) and Listeners inside existing implementations of all open-source IntelliJ Platform plugins published on JetBrains Marketplace. You can navigate directly to the source files to find inspiration when implementing your own extensions and listeners for IntelliJ-based IDEs.
3.3 Browse Available References
The IntelliJ Platform SDK Documentation should always be the first resource you check for information. Here is a condensed list you can use for further reference:
Section on exploring module and plugin APIs.
List of notable and incompatible API changes.